How to show images in gridview from sdcard in android
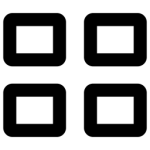
You must be wondering how to show images in gridview from sdcard in android If you are developing an Android app and want to display images from the SD card in a user-friendly format? then .
The GridView layout can provide a great way for users to interact with images stored on their device. GridView displays items in two-dimensional grids which allows users to scroll through data more quickly and efficiently than with ListViews. With GridView, it’s easier to understand the context of each image displayed on the screen.
In this article, we will show you how to use GridViews in your Android app to display images from an SD card. We will demonstrate how to configure the layout and how perform operations like adding, deleting and updating images within GridView.
Create a GridView Layout
The first step is to create a grid view using the GridView element in your layout file. Grid views can be customized by setting attributes such as the number of columns, column widths, and column spacings.
you need to get the list of image file paths from the SD card. You can use the Environment.getExternalStorageDirectory() method to get the root directory of the SD card, and then use the File class to traverse the directory structure and get a list of image file paths. Here’s an example of how to do this:
// Get the root directory of the SD card
File sdCardRoot = Environment.getExternalStorageDirectory();
// Get a list of image file paths
List<String> imagePaths = new ArrayList<>();
for (File f : sdCardRoot.listFiles()) {
if (f.isFile() && f.getName().endsWith(".jpg")) {
imagePaths.add(f.getAbsolutePath());
}
}
Set the Adapter
A GridView adapter must be set once the layout has been created. An SD card list of images should be initialized with this adapter so it can provide data to the GridView. To populate the GridView with all images from the SD card, you can use a CursorAdapter.
public class ImageAdapter extends BaseAdapter {
private Context context;
private List<String> imagePaths;
public ImageAdapter(Context context, List<String> imagePaths) {
this.context = context;
this.imagePaths = imagePaths;
}
@Override
public int getCount() {
return imagePaths.size();
}
@Override
public Object getItem(int position) {
return imagePaths.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ImageView imageView;
if (convertView == null) {
imageView = new ImageView(context);
imageView.setLayoutParams(new GridView.LayoutParams(200, 200));
imageView.setScaleType(ImageView.ScaleType.CENTER_CROP);
imageView.setPadding(8, 8, 8, 8);
} else {
imageView = (ImageView) convertView;
}
// Load the image from the file path into the ImageView
Bitmap bitmap = BitmapFactory.decodeFile(imagePaths.get(position));
imageView.setImageBitmap(bitmap);
return imageView;
}
}
Set the adapter using the setAdapter method
A custom adapter can be used to display images from an SD card in a grid view as shown below:
GridView gridView = findViewById(R.id.gridview);
gridView.setAdapter(new ImageAdapter(this, imagePaths));
Advantages of using a GridView
- GridViews enable you to display a large number of items in a grid layout, with each item in its own cell. A compact, organized display of data can be useful if you have a lot of data.
- In terms of displaying a large number of items, a GridView is more efficient than a ListView. GridView loads only the items that are currently visible on screen, while the ListView loads all items at once. Performance and responsiveness of your app can be improved by doing this.
- By using a custom adapter, GridView cells can be customized. With this feature, you can specify the layout and content of each cell, allowing you to display your data in a variety of ways.
- GridViews allow various interactions, such as clicking an item to perform an action or long pressing an item to display a context menu. By doing this, users can easily interact with your app and access the data they need.
- Implementing and using a GridView is easy. Many developers find it convenient because it requires only a few lines of code to set up and display data.